記事のターゲット
- Springについてある程度理解している方で、テンプレートにThymeleafを使用している方。
環境
- Eclipse pleiades-2019-12-java
- Spring 2.3.2.RELEASE
- thymeleaf-spring4 3.0.0.RELEASE
使用方法
まず今回例で使用するのは、スケジュール管理用のアプリで、Form画面で時刻を入力し確認画面へ渡すプログラムです。 適当に作ったものなので画面の出来は気にしないでください。
step
1Formクラス作成
package example.application.form.schedule;
import java.io.Serializable;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import org.springframework.format.annotation.DateTimeFormat;
import example.application.form.user.UserForm;
public class ScheduleForm implements Serializable{
private int id;
private String title;
private String content;
@DateTimeFormat(pattern = "yyyy-MM-dd")
private LocalDate startDate;
private LocalTime startTime;
@DateTimeFormat(pattern = "yyyy-MM-dd")
private LocalDate endDate;
private LocalTime endTime;
private LocalDateTime createdAt;
private LocalDateTime updatedAt;
private UserForm userForm;
Form画面で日付や時刻のフィールドを作成したらFormatしてあげる必要があります。今回は日付と時刻を分けているためLocalDateとLocalTimeに分けていますがLocalDateTimeでも同じことができます。
step
2入力画面作成
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>スケジュール作成</title>
<meta charset="UTF-8" />
<script th:src="@{/js/scheduleCreate.js}"></script>
</head>
<body>
<h1>スケジュール作成</h1>
<p>下のやつ入力して</p>
<form th:action="@{/schedule/confirm}" th:object="${scheduleSessionForm}"
method="post">
<p class="schedule_title">タイトル</p>
<input type="text" name="title" th:field="*{scheduleForm.title}">
<p class="schedule_content">詳細</p>
<input type="text" name="content" th:field="*{scheduleForm.content}">
<p class="schedule_start">開始日</p>
<input type="date" name="startDate" th:field="*{scheduleForm.startDate}">
<p class="schedule_start">開始時間</p>
<input type="time" name="startTime" th:field="*{scheduleForm.startTime}">
<p class="schedule_end">終了日</p>
<input type="date" name="endDate" th:field="*{scheduleForm.endDate}">
<p class="schedule_start">終了時間</p>
<input type="time" name="endTime" th:field="*{scheduleForm.endTime}">
<p><input type="submit" value="次へ" class="btn"></p>
</form>
</body>
</html>
JSPでもThymeleafでも共通なのが、<form>タグで囲んだ部分を入力部分にすることです。Controllerに送ったときにきちんと格納されるように、Formクラスで指定したフィールドと対応する型をinput typeに指定しましょう。
ThemeleafではJSPと違いthタグでいろいろ済ますので、今回使用しているタグを下にまとめておきます。
ソースで使用しているthタグ | 説明 |
xmlns:th | Thymeleaf使いますよという宣言 |
th:src | 今回はJSファイルの読み込みに使っています |
th:action | ControllerのRequestMappingと一致させるパス |
th:object | Formタグ内で使用する、Modelに格納されているプロパティ名指定 |
th:field | th:objectで指定したプロパティのフィールドを出力する |
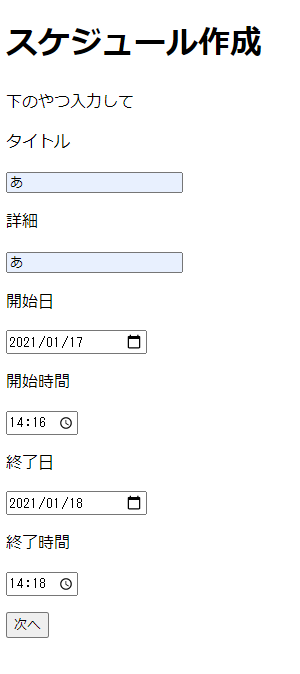
step
3Controller作成
package example.application.controller.schedule;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.SessionAttributes;
import example.application.form.schedule.ScheduleForm;
import example.application.form.schedule.ScheduleSessionForm;
@Controller
@SessionAttributes(names = "scheduleSessionForm")
public class ScheduleController {
private static final String FORM_NAME = "scheduleSessionForm";
@ModelAttribute(value = "scheduleSessionForm")
public ScheduleSessionForm scheduleSessionForm() {
return new ScheduleSessionForm();
}
//トップページとかいろいろ省略
@RequestMapping(path = "schedule/create", method = RequestMethod.GET)
//@ModelAttributeで指定したFormに先ほど入力した値が自動的に格納される
public String createSchedulePage(@ModelAttribute(FORM_NAME)ScheduleSessionForm scheduleSessionForm,Model model) {
scheduleSessionForm.setScheduleForm(new ScheduleForm());
model.addAttribute("scheduleSessionForm", scheduleSessionForm);
return "schedule/schedule-create";
}
@RequestMapping(path = "schedule/confirm", method = RequestMethod.POST)
public String newSchedulePage(@ModelAttribute(FORM_NAME)ScheduleSessionForm scheduleSessionForm,Model model) {
model.addAttribute("scheduleSessionForm", scheduleSessionForm);
return "schedule/schedule-confirm";
}
}
送られてきたFormをプロパティに格納して確認画面へ渡すします。
step
4確認画面作成
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>スケジュール確認</title>
<meta charset="UTF-8" />
<script th:src="@{/js/scheduleCreate.js}"></script>
</head>
<body>
<h1>スケジュール作成</h1>
<p>下のやつ確認して</p>
<form th:action="@{/schedule/new}" th:object="${scheduleSessionForm}"
method="post">
<p class="schedule_title">タイトル</p>
<span th:text="*{scheduleForm.title}"></span>
<p class="schedule_content">詳細</p>
<span th:text="*{scheduleForm.content}"></span>
<p class="schedule_start">開始日</p>
<span th:text="*{scheduleForm.startDate}"></span>
<p class="schedule_start">開始時間</p>
<span th:text="*{scheduleForm.startTime}"></span>
<p class="schedule_end">終了日</p>
<span th:text="*{scheduleForm.endDate}"></span>
<p class="schedule_start">終了時間</p>
<span th:text="*{scheduleForm.endTime}"></span>
<p><input type="button" th:value="*{btnPrev}" class="btn"></p>
<p><input type="submit" th:value="*{btnExec}" class="btn"></p>
</form>
</body>
</html>
ソースで使用しているthタグ | 説明 |
th:text | Modelに格納されているフィールドを出力させるタグ |
th:value | HTMLのvalue部分にフィールド等を使用したい場合に使う。今回は省いていますがボタンの名前を動的に変化させる場合に使用します。 |
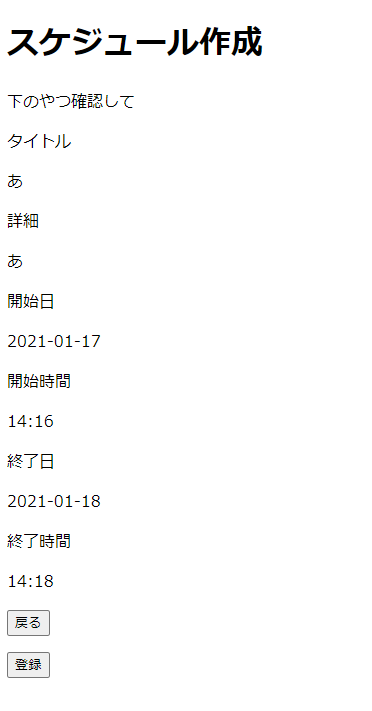
先ほど入力したものが指定したフォーマットに則って表示されています。
まとめ
今回はThymeleafでForm画面を作成する流れで時刻の扱いを紹介しました。例の中では日付と時刻を別々にしてサーバー側の処理の柔軟性を上げていますが、日付と時刻を同時に入力できる<input type = "datetime-local">という記述方法もあります。Thymeleafはコーダーとデザイナーが同時に仕事しやすいとして最近の主流になっているので使えるようになっていると便利だと思います。
今回使用したタグまとめ
ソースで使用しているthタグ | 説明 |
xmlns:th | Thymeleaf使いますよという宣言 |
th:src | 今回はJSファイルの読み込みに使っています |
th:action | ControllerのRequestMappingと一致させるパス |
th:object | Formタグ内で使用する、Modelに格納されているプロパティ名指定 |
th:field | th:objectで指定したプロパティのフィールドを出力する |
th:text | Modelに格納されているフィールドを出力させるタグ |
th:value | HTMLのvalue部分にフィールド等を使用したい場合に使う。今回は省いていますがボタンの名前を動的に変化させる場合に使用します。 |